If you are planning to start developing a web application, website, or a mobile application, then one of the decisions you need to make is what programming languages and tools are you going to use in the development process.
And if we are talking mainly about the web, then preferably you need to pick a tool that will help you develop backend and frontend together with ease.
In the last article we have talked about different types Frontend Development For Beginners
In this article we will introduce Laravel to you as one of the best options you have.
What is Laravel?
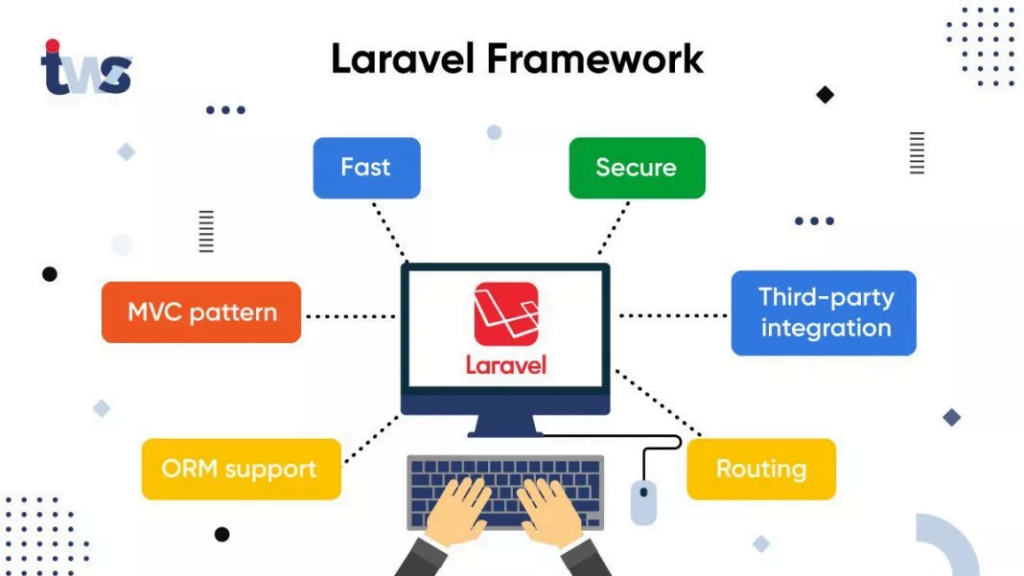
Laravel is not a programming language. But a framework based on the PHP language. It is used to build high-end web applications and sites. It was developed by Taylor Otwell in 2011, and is now one of the most popular open-source PHP-based frameworks.
Laravel Features
Laravel is a very rich framework, it has a lot of features that made it very popular among web developers, either in the backend or frontend as well. If you are familiar with PHP development already, Laravel will make your life so much easier. And if you are a beginner, starting with Laravel means you don’t have the face the complexities of pure PHP.
So let’s explore the main features of Laravel.
MVC model components
Laravel follows the MVC (Model-View-Controller) architecture pattern, which very efficiently relates the user interface to the underlying data models, and separates the concerns and responsibilities of codes and developers into 3 different parts.
MVC is divided into three main components, identified by the letters of the acronym:
- M: for Model, which is a class that represents your data. And deals with the database. For example if your application or site has users, then you will have a User model. Which will have the properties of this user like name, age, address.
- V: for View, which is mainly related to the User Interface (UI), in the web world this means it deals with your frontend components like HTML, CSS, JavaScript, and everything your users see in the browser. It also handles the communication between the users and the Controller.
- C: for Controller, which is the central unit for the MVC architecture. It is the main request handler, which links the user to the system. It is simply the middle-man that takes the data from the Model and sends it to the View.
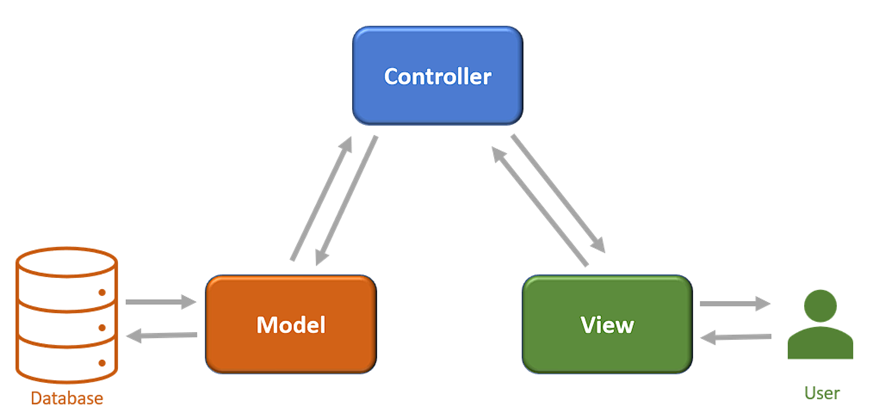
The main benefits for MVC architecture, and hence Laravel as well, is it makes it easier to organize large size applications, as there is separation of code among the three components. This means that the application and its components will be easier to modify and update, and faster to develop.
Separating the Views from the Models and Controllers means you can reuse your code, either in different parts of the same application, or even in a completely different application. Just by replacing the View files with different ones that have different designs you can have a completely different UI. You can have multiple themes for your websites. Or even deliver the same application for different clients, where every client has their own visuals and interface.
Query Builder and ORM
Laravel includes an Object Relational Mapper (ORM) called Eloquent, that makes it very easy and enjoyable to interact with your database. Each database table corresponds to a Model that is used to interact with that table. Eloquent also helps with database functionalities like inserting, updating and deleting records.
Using Eloquent means you will perform all your query using your models instead of querying the database directly. This means your code will be much simpler and easier to read. Take his example, suppose you want to find the user with id=1 in your database, normally you will do something like this:
$user = DB::table('users')->where('id', '1')->first();
However with Eloquent, you can just use this:
$user = App\User::find(1);
Much simpler, huh? Sure. Eloquent works like magic, once you get used to it, you cannot go back to using the traditional queries, it will feel outdated. In the following articles in this series, we will dive deeper into Eloquent and how to use it efficiently.
Routing
One of the great features of Laravel is that it provides a very flexible routing system. Routing system in Laravel helps you to easily route your application requests to the appropriate controller. Laravel routes can be named, so you can simply use the route name in your code to easily identify the route. It allows grouping routes, so a group of routes that handle a certain model for example and requires that same authentication can be easily handled in a simple manner.
This is an example of a named route:
Route::get(
'/user/profile',
[UserProfileController::class, 'show']
)->name('profile');
And this is how you group routes that use the same controller for simpler code:
Route::controller(OrderController::class)->group(function () {
Route::get('/orders/{id}', 'show');
Route::post('/orders', 'store');
});
Laravel Routing system also supports inserting parameters in your code, both required and optional parameters for more flexibility, check this example:
Route::get('/posts/{post}/comments/{comment}', function ($postId, $commentId) {
// post and comment parameters here are mandatory
});
Route::get('/user/{name?}', function ($name = 'John') {
// the name parameter is optional here, we can provide a default value if needed
return $name;
});
There are more and more features in the Laravel Routing system that will dedicate a full article in this series to the routing system.
Blade Templates
Blade is a very simple and powerful templating engine that is included with Laravel. It makes writing your views very simple. The Blade engine provides its own syntax and structure, such as conditional statements and loops, and it allows using nested template inheritance. And it allows using plain PHP code inside the templates as well.
The Blade syntax is very clean and easy to handle and understand, Blade syntax is compiled into PHP code and then cached, which means it provides better web server performance than using plain PHP alone.
Blade templates usually reside in the views folder of your Laravel project. And they can be identified by their extension “.blade.php”, you can organize the templates in folders that follows your model structure for easier understanding.
Here is an example of a simple blade template:
@if (count($students) > 1)
<ul>
@foreach($students as $student)
<li>{{$students}}<li>
@endforeach
</ul>
@endif
As you can see, the template is very simple and self-explanatory, and this is the power of Blade.
Authentication
Most Web applications provide a way for users to authenticate and login, this feature is usually complex and risky. If done wrong, the security of your application and the data of your users can be at a big risk of being leaked or manipulated.
This is why Laravel helps you offload the burden of authentication off your shoulders, and provides guards and providers that can handle the authentication part of your application with minimal effort from you. Guards define how users are authenticated for each request. Providers define how users are retrieved from your database.
Laravel also provides two packages that can handle your API authentication needs, Passport and Sanctum. Passport is an OAuth2 authentication provider that allows you to issue different types of tokens. However OAuth2 is complex and confusing, this is why they provided Sanctum as a simpler alternative that can handle both web requests from browsers and API requests via tokens.
Handling authentication in your case is highly integrated in Laravel, it is a simple as writing 2 words of code like Auth::check(), here are some sample codes that shows basic authentication in Laravel
use Illuminate\Support\Facades\Auth;
// get the currently authenticated user...
$user = Auth::user();
// get the currently authenticated user's ID...
$id = Auth::id();
if (Auth::check()) {
// if the user is correctly authenticated, then do this...
}
Route::get('/products, function () {
// Only authenticated users may access this route...
})->middleware('auth');
Just as simple as that. Your application and user’s data are safe and secure.
Sessions
Sessions are used to store user’s information across different pages and requests in your application. Laravel conveniently provides several storage drivers to store your application’s session data, here are the list of session providers as listed in Laravel documentation:
- file – sessions are stored in storage/framework/sessions.
- cookie – sessions are stored in secure, encrypted cookies.
- database – sessions are stored in a relational database.
- memcached / redis – sessions are stored in one of these fast, cache based stores.
- dynamodb – sessions are stored in AWS DynamoDB.
- array – sessions are stored in a PHP array and will not be persisted.
The Files driver is the default for Laravel, but this can easily be changed in your application settings, by simply changing it in config/session.php.
Using the session environment in Laravel makes it easy to temporarily store and retrieve data in your application, here is a sample code
// store name variable using the global "session" helper...
session(['name' => 'John Smith']);
// Retrieve the name from the session...
$name = session('name', 'Default Value');
Data Validation
When a user submits data to your website, it is very important to check the validity of this data, for example his email must be in the correct format, phone numbers consists only of numbers and with specific length in some cases, password needs to have specific strength measures and need to be confirmed by entering it twice, and other validation rules for different data types.
Laravel provides its own validation methods to help you validate incoming data. When your application receives an HTTP request from the user you pass this data through the validation rules, and if they match you can proceed forward, otherwise return to the user with specific error messages.
Here is a sample validation code you will typically use in Laravel apps.
public function store(Request $request)
{
$validated = $request->validate([
'email' => 'required|email',
'username' => 'required|unique:users|max:25',
'password' => 'required|confirmed|min:8',
]);
// If inputs are validated, proceed with registration.
}
In this code, we require the user to enter his email, which must be formatted in the correct way. Also a username is required, it must be unique in our users database to avoid repetition, and at last a password that must be at least 8 characters long, and confirmed by entering it twice.
Laravel Requirements
Now you have a good understanding of what Laravel is, and the great features it offers to make developing your website much easier. So what do you need to start developing your website with Laravel?
1. Basic Understanding of PHP
As explained earlier in this article, Laravel is not a programming language by itself, however it’s a framework based on the PHP language. In order to develop with Laravel you need to know your way around with PHP, and for sure SW development basics.
2. A Web Server
Laravel is based on PHP, which needs a web server to run. You cannot just pick up your PC or Mac and start developing with PHP. You need to prepare an environment for it to run. You have the following options:
- Use Docker Container (Windows, MacOS & Linux)
- Windows WSL2
- Develop on Linux with the web server installed
- Install a Webserver on your computer like WAMP for Windows or MAMP for Mac.
3. MySQL
Laravel needs a MySQL database engine to build its database. So you need to have it installed in the environment you prepared in the previous step. If you go with the last option (WAMP server), it already has MySQL included.
4. Suitable IDE
The IDE is the program that you use to write your code. Having a good IDE will help you configure your work environment easily and will increase your productivity. There are lots of code editors out there to choose from, but we will suggest 2 IDEs, we think are the best with ability to add extensions dedicated to PHP and Laravel
Developed by Microsoft , and is considered one of the best free development IDEs out there, It is free and very powerful. It has integrated Git control and terminal. It also has a very large plugin system that makes your life easier with code snippets and code suggestions.
PHPStorm is the perfect tool to work with Laravel and PHP in general. It analyzes your code structure and provides suggestions, completion, refactoring and error prevention. Like Visual Studio Code it also has a very large plugin system, with many plugins dedicated to Laravel Development.
Conclusion
Now you are ready to start development with Laravel. The first step is to install the Laravel framework itself and start your first project. And this is exactly what we will discuss in the next article, so follow us.