Laravel is a very feature-rich and powerful platform, it includes a lot of libraries that makes your development process easy and productive. However if there is a certain feature of Laravel that can feel joyful to use, it would be Blade View templates for sure.
Blade View is a simple yet powerful templating engine that is included with Laravel. It uses simple and easy to understand directives that look similar to PHP code to integrate your Laravel code with HTML. They use the .blade.php extension and are typically located in the resources/views directory.
In the earlier article we have discussed about Ultimate Beginners Guide With Laravel
What Is a Templating Engine?
Templating engines are usually used when you want to build web applications that are split into different components, they provide easy ways for backend data to communicate with frontend views. A frontend view is what ultimately displays the user interface using HTML, and the backend processes and provides the actual data. This helps you by eliminating the need to write backend functions just to display data inside your views.
When you use a template engine to build a server-side application, the template engine will replace the variables in a template file with actual values passed to it from the controllers, and display this value to the end user. This method makes it easier to build our application quickly.
Blade is Laravel’s solution for template engine, we used it briefly in our previous articles in this series. But in this article we will explore it in more detail.
Displaying Data
The most basic functionality of Blade View is to display data passed to it in variables, and combine them with the HTML of your web page. This is done by wrapping the variable in double curly braces. For example if you have a variable $name passed to a blade view, you can use it like this
<p>Welcome to Laravel, {{$name}}</p>
You can also display the results of PHP functions by wrapping them in the double curly braces, for example
The time now is {{ time() }}.
Layouts using yield and section
You can create an inherited template for your website, by creating a master layout that will be used across the website. This template will be used by all your web pages to inject contents into this layout. Take a look at this layout for example
<html>
<head>
<title>App Name - @yield('title')</title>
</head>
<body>
@section('sidebar')
This is the master sidebar.
@show
<div class="container">
@yield('content', 'Default content')
</div>
</body>
</html>
This is a very basic HTML code that has all the typical web page components. As you can see there are 2 main directives here. @yield and @section. The first directive @yeild is used for content that will be updated depending on the page, in this example each page will provide its own title and contents, so we used yield directive for this. The @section directive defines a section of the content that will be provided by the layout view.
The layout view will not be used by itself ever, however it will be inherited by other child views, so let’s build a child view that will use this layout and see how it will look like
@extends('layout')
@section('title', 'Page Title')
@section('sidebar')
@parent
<p>Add this text to the master sidebar from the layout.</p>
@endsection
@section('content')
<p>This is the content of this page.</p>
@endsection
The first line in this child view defines the master layout that it will use, using the @extends directive, which has the name of the layout file. Then we use the @section to define the title, which will be displayed in the layout by replacing the @yield directive with the same name.
The sidebar section uses the @parent directive, which means it will append that section to the layout rather than overwriting it, so it will add its own content to the sidebar section in the layout.
And at last the content section will replace the @yeild with the same name in the layout. Note that this @yield in the layout has a default value, so if the child view doesn’t provide a @section for it, the default value will be displayed instead.
Conditional Statements
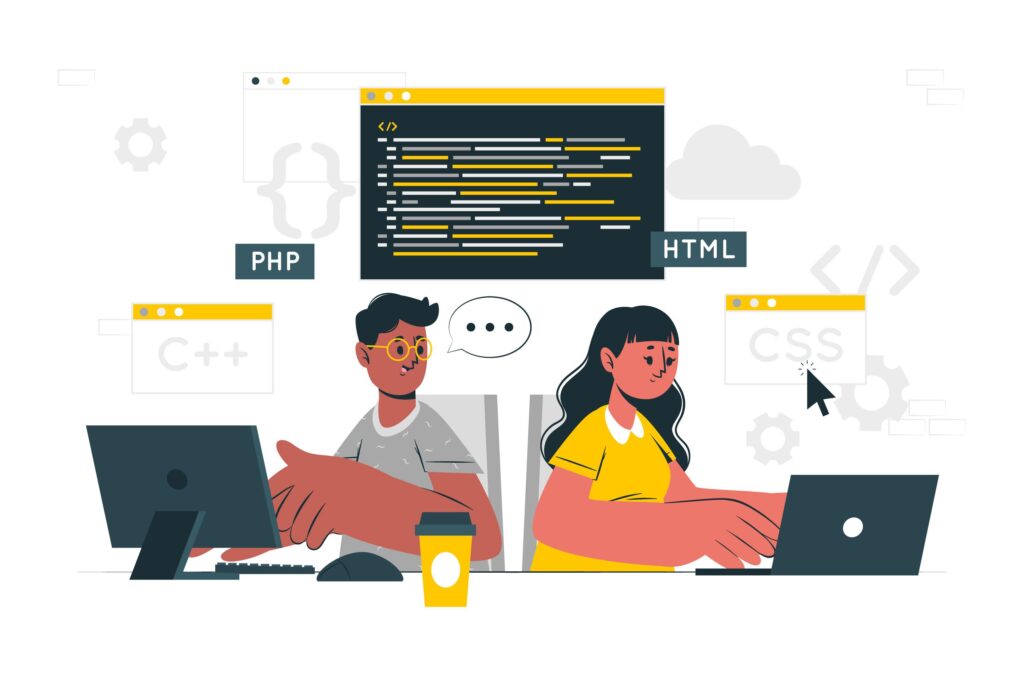
Conditional statements are used through the various programming languages to decide what to do when given some conditions. Blade View also has its own conditional statements in the form of directives as usual. The most used conditional directive is @if statement which is very identical to its PHP counterpart
@if ($role == ‘admin’)
Welcome To the Admin dashboard!
@elseif ($role == ‘user’)
Welcome to our website
@else
Please login to view the content.
@endif
Blade View also has an @unless directive which is similar to saying if not
@unless ($role == ‘editor’)
You are not allowed to edit this content.
@endunless
You can also check if the user is authenticated in the website or not
@auth('admin')
// The user is authenticated...
@endauth
@guest('admin')
// The user is not authenticated...
@endguest
Switch Statements
Switch statements provide you with means to show different content based on different conditions, similar to @if statement but with ability to add several conditions
@switch($role)
@case(‘admin’)
Welcome to the Admin dashboard.
@break
@case(‘user’)
Welcome to our website
@break
@default
Please login to view the content.
@endswitch
Loops
In many scenarios, you will have an array of items and wish to list them in your view in a table or a list. Iterating through this array is called looping. Similar to PHP Blade provide @for loop and @foreach
<ul>
@for ($id = 0; $id < 10; $id++)
<li>{{$book[id]->name}} </li>
@endfor
</ul>
<table>
<tbody>
@foreach ($books as $book)
<tr>
<td>{{$book->id}}</td>
<td>{{$book->name}}</td>
<td>{{$book->author}}</td>
<td>{{$book->isbn}}</td>
</tr>
@endforeach
</tbody>
</table>
What if the array is empty, and you want to have the option to display a specific message in this case, instead of showing a table of items for example, in this case you can use @forelse
<table>
<tbody>
@forelse ($books as $book)
<tr>
<td>{{$book->id}}</td>
<td>{{$book->name}}</td>
<td>{{$book->author}}</td>
<td>{{$book->isbn}}</td>
</tr>
@empty
<tr> There is no book in this section </tr>
@endforelse
</tbody>
</table>
Partials (Subviews)
One of the most common features of Blade View that you will use, is partials or subviews. This means you can divide your webpage into smaller components, some of them can be recyclable through the application like the header and footer of the pages. And some partials might be used only for this page, but we divide them into smaller components for easier handling.
Take a look at the below diagram, this is a typical web page that consists of a header, sidebar, contents and footer, in this example we created a blade view for each of these components, then we added them all to the main blade view that will be shown to the user.
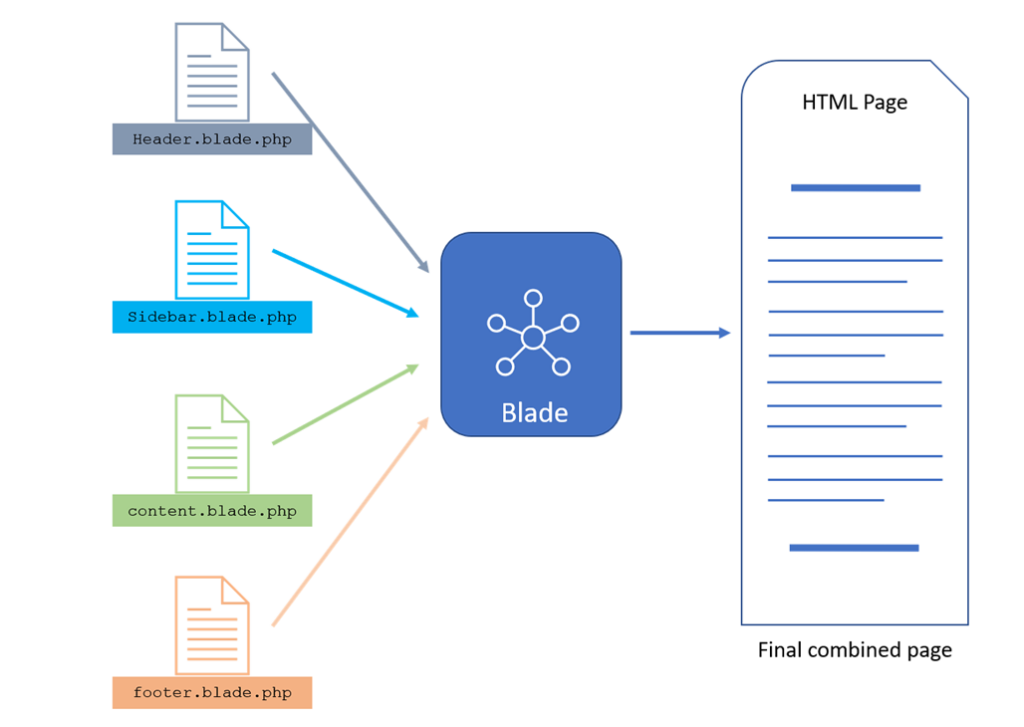
This web view will have this simple code to include all the 4 subviews and display them as one file.
@include('header.blade.php')
@include('sidebar.blade.php')
@include('content.blade.php')
@include('footer.blade.php')
You can pass parameters to the included subviews as well
@include('view.name', ['name' => 'John Smith'])
Or you can include a view only if a certain condition is true
@includeWhen($role == ‘admin’, 'view.admin', ['status' => 'Logged In'])
If you have a certain view that needs to be repeated for several items in an array, you can use @each directive, you can also provide a view for the case that there are items in the array to display instead.
@each('view.name', $books, 'book', 'view.empty')
Forms
Blade View has special directives to handle HTML forms, including directives for security, submission methods, and validation.
One of the most important directives to know about, that must be included in all forms is @csrf, this directive will include a hidden token in the form. This is used to protect your website from CSRF (Cross-Site Request Forgery) which is a web security vulnerability that allows an attacker to induce users to perform actions that they do not intend to perform. Here is how to use it.
<form action="/update" method="POST" >
@csrf
...
</form>
When a form is created, you need to decide what is the method it uses, HTML allows only GET and POST methods. Laravel however can accept PUT, PATCH, and DELETE, to solve this, Blade View uses the directive @method, which adds a hidden _method field that submits that actual method within a POST request.
<form action="/update" method="POST">
@csrf
@method('PATCH')
...
</form>
<form action="/delete" method="POST">
@method('DELETE')
...
</form>
Form validation is also an important issue to handle in your frontend. As discussed in previous articles in this series, validation is done in controllers, and if validation fails, the controller provides error messages to your view, which you need to check and display to the user so that he can know what was wrong in his inputs. This is done using the @error directive, which is a sort of a condition that becomes true if there is an error with the defined name in the error bag.
<label for="name">User Name</label>
<input id="name" type="text" class="@error('name') is-invalid @enderror">
@error('name')
<div class="alert alert-danger">{{ $message }}</div>
@enderror
In the above code, if the user submits a user name that doesn’t comply with the validation rules, there will be an error coming back from the controller in the bag with the key ‘name’, and in this case the class is-invalid will be applied to the input, and the div with alert message will be displayed as well. You can also use @else directive to provide a class or any other tags you want in case there is no error.
<label for="name">User Name</label>
<input id="name" type="text"
class="@error('name') is-invalid @else is-valid @enderror">
@error('name')
<div class="alert alert-danger">{{ $message }}</div>
@error
<div class="alert alert-success">User name accepted</div>
@enderror
Stacks
Blade View provides you with the ability to push content that can be rendered somewhere else in another view or layout. This feature is called stacks. Using stacks can be very useful to add specific JavaScript or CSS libraries that are required by the child view and not defined in the parent or layout view
<!-- This part is in the parent view where the stacks will be added -->
<head>
@stack('scripts')
</head>
<!-- This part is in the child view That will push the stack -->
@push('scripts')
<script src="/script.js"></script>
@endpush
Adding Custom Blade View directives
Finally, If you need to have a specific functionality that is not provided by Blade View directives, you can define your own custom directives as well. These directives need to be defined in the app/Providers/AppServiceProvider.php file, in the boot()method as follows
<?php
namespace App\Providers;
use Illuminate\Support\Facades\Blade;
use Illuminate\Support\ServiceProvider;
class AppServiceProvider extends ServiceProvider
{
/**
* Register any application services.
*
* @return void
*/
public function register()
{
//
}
/**
* Bootstrap any application services.
*
* @return void
*/
public function boot()
{
Blade::directive('add', function ($num1, $num2) {
return "<?php echo ($num1 + $num2); ?>";
});
}
}
In the above example we defined a directive that when used in a blade View, it will add 2 numbers and display the result in the final view
<p> The result of adding 3 and 4 is @add(3,4)</p>
Conclusion
Blade is a very rich templating engine that comes included freely in the Laravel framework, mastering Blade can save you a lot of time, effort and code. There are also some more advanced Blade directives that you can learn about in the official Laravel documentation here.
In the coming articles we will continue exploring Laravel features and explain more of them, so follow us.